How to prevent HTML form input autofill?
The HTML form inputs can have the autocomplete="off"
attribute set to prevent automatically filling of such input.
However most browsers tend to ignore this attribute, due to
annoying designs of many web applications, which are forbidding
saving passwords on logins forms. That's my opinion based on
development experience. However, there might be cases where
automatically completing inputs - especially passwords - will
cause issues.
Some example cases I've stumbled upon:
- When setting new password will change the old one
- When secrets need to be provided for external services, for instance for OAuth2 providers
The workaround to enforce autocomplete to be off is to use
readonly
attribute. Then the form input is read
only, well the browser cannot fill it. The idea is to create read
only input, then remove this attribute on page load. This could
almost work, however we also need slight delay, as the
autocomplete mechanism is executed at very end of page rendering.
Please note that the solution presented here will allow autocomplete, but it will not be preformed automatically on page load
Example implementation
First of all, let's create form input with extra CSS class to select it with jQuery:
<input autocomplete="off" class="autocomplete-off" name="password" type="password" readonly="true">
With small piece of JavaScript we remove readonly
attribute after slight delay:
$(document).ready(function() {
var off = function(){
var fields = $('.autocomplete-off');
fields.removeAttr('readonly');
};
setTimeout(off, 50);
});
Footnotes
This solution might not always work, as browsers tend to be smarter and smarter. The password suggestion menu will still be shown when input is focused. The solution presented here is to allow user to decide if he want to fill input or not - that is if someone want to use autocomplete - he still can. The autocomplete feature is a kind of silent war between users who just want to have better experience, browsers developers who try to automate things and web applications developers who just want to have some inputs to be manually completed.
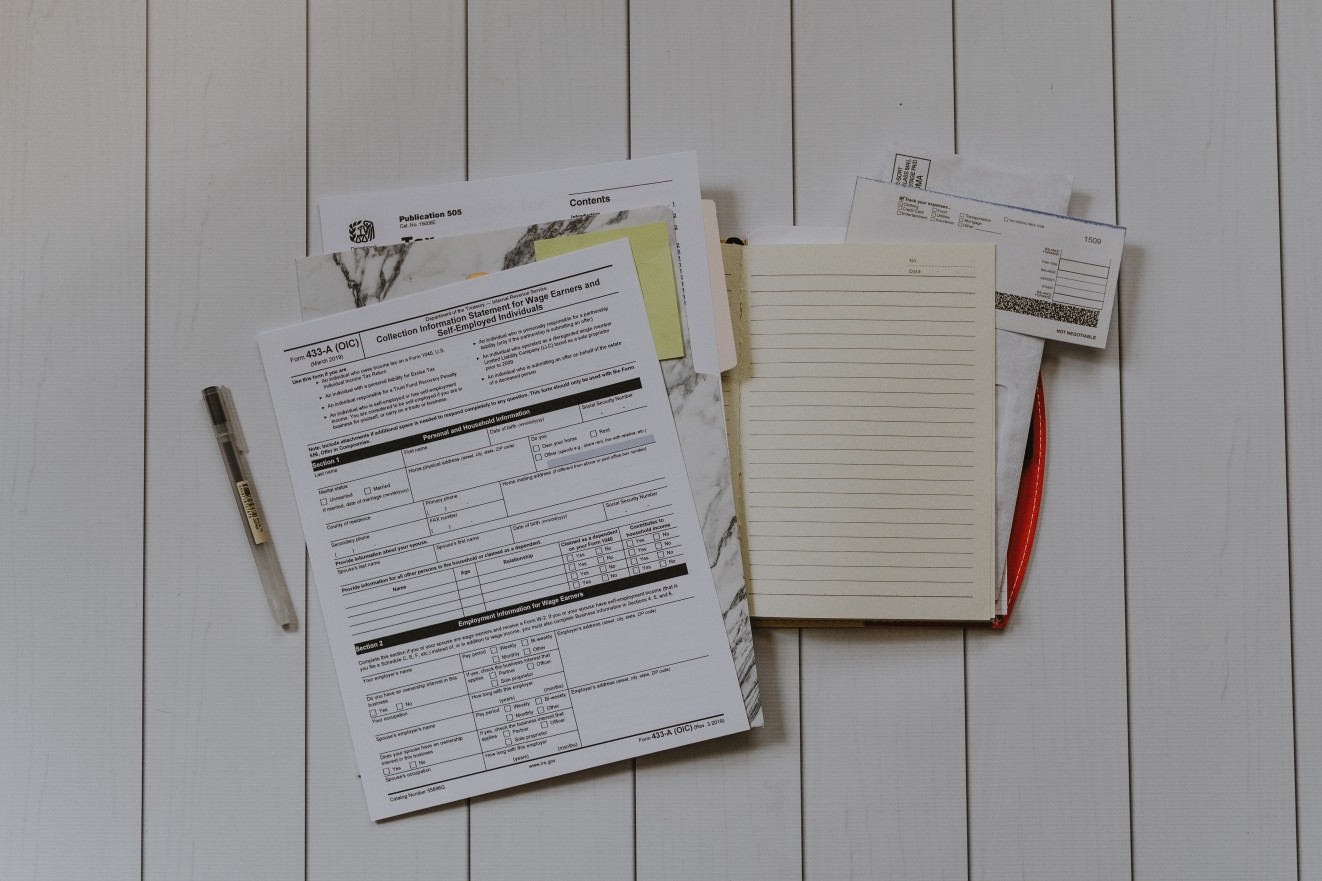